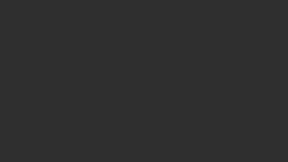
Diminish
Slip and slide across the floor in this physics-based game and try to eat the green bubbles to increase your size while you shrink over time. Use the up and down arrows to move forward and backward while using the right and left arrows to rotate and steer.
Code:
//Configure app for landscape game mode.
cfg.Game, cfg.Landscape
//Handle game loading.
function OnLoad()
{
//Enable physics & enclose scene with walls.
//Note: zero gravity because it is slippery!
gfx.AddPhysics( 0 )
gfx.Enclose( -1, "left,top,right,bottom", 0.1,0.1,0.1, 0.05 )
//Create background.
floor = gfx.CreateBackground( "Img/Floor2.png" )
//Create sprites.
blob = gfx.CreateSprite( "Img/blob_1000x1500x5.png", "blobs" )
food = gfx.CreateSprite( "Img/Food.png", "foods" )
gameover = gfx.CreateSprite( "Img/gameoverend.png" )
//Create sounds.
//(Sounds from www.zapsplat.com)
soundEat= gfx.CreateSound( "Snd/pop.mp3" )
soundDiminish = gfx.CreateSound( "Snd/diminish.mp3" )
soundMusic= gfx.CreateSound( "Snd/music1.mp3" )
}
//Called when game has loaded.
function OnReady()
{
//Set background.
gfx.AddBackground( floor )
//Add game objects to screen.
AddBlob()
AddButtons()
//Add more food every so often
setInterval( AddFood, 3000 + 3000*Math.random() )
//Start animating
gfx.Animate( OnAnimate )
//Play Music
soundMusic.Play( true, 500 )
}
//Update game objects.
function OnAnimate( time, timeDiff )
{
//Decrease blob size over time
blob.width = blob.width - 0.0001 ;
blob.height = blob.height - 0.0001 ;
//When blob size reaches 0, game over.
if( blob.width < 0)
{
//Play game over sound.
soundDiminish.Play() ;
//Show game over screen
gfx.AddSprite( gameover, 0.22, 0.4, 0.5, 0.25 ) ;
gfx.Pause() ;
}
}
//Add a new blob to screen.
function AddBlob()
{
//Add blob image to center of screen & show frame 4.
gfx.AddSprite( blob, 0.45, 0.5, 0.1 )
blob.Goto( 4 )
//Set physics properties for blob.
//group, type, density, bounce, friction etc.
blob.SetPhysics( -2, "dynamic", 0.9, 0.9, 0.3, 0.3, 0.3 )
blob.SetShape( "rect", 0.7, 0.7 )
}
//Add food image to screen, with physics and animation.
function AddFood()
{
//Add a new food image to screen at random x location.
food = gfx.CreateSprite( "Img/Food.png", "foods" )
var size = 0.1+0.1*Math.random()
gfx.AddSprite( food, Math.random()*0.7, -0.3, size )
//Set physics properties for food.
food.SetPhysics( -1, "dynamic", 0.9, 0.9, 0.9 )
food.SetShape( "round", 0.5 )
food.SetVelocity( -0.01+0.02*Math.random(), 0.02/size, -0.05+0.1*Math.random()/size )
}
//Add control buttons for mobile.
function AddButtons()
{
//Left stick
var stickSize = 0.08
leftStick = gfx.CreateCircle( stickSize, 0x04defb )
gfx.AddGraphic( leftStick, 0.005, 1-leftStick.height*2 )
leftStick.alpha = 0.3
//Right stick
rightStick = gfx.CreateCircle( stickSize, 0x04defb )
gfx.AddGraphic( rightStick, leftStick.width*1.5, 1-rightStick.height*2 )
rightStick.alpha = 0.3
//Forward stick
fwdStick = gfx.CreateCircle( stickSize, 0x3bf906 )
gfx.AddGraphic( fwdStick, leftStick.width*0.75, 1-fwdStick.height*3+0.02 )
fwdStick.alpha = 0.3
//Reverse stick
revStick = gfx.CreateCircle( stickSize, 0x3bf906 )
gfx.AddGraphic( revStick, leftStick.width*0.75, 1-revStick.height-0.02 )
revStick.alpha = 0.3
}
//Handle key presses and screen touches.
function OnControl( touchState, touchX, touchY, keyState, key )
{
//Check for arrow keys or joystick touches
if( key=="ArrowLeft" || leftStick.Contains(touchX,touchY) )
{
blob.AddVelocity( 0, 0, -0.01 )
blob.Goto(2)
}
else if( key=="ArrowRight" || rightStick.Contains(touchX,touchY) )
{
blob.AddVelocity( 0, 0, 0.01 )
blob.Goto(4)
}
else if( key=="ArrowUp" || fwdStick.Contains(touchX,touchY) )
{
blob.AddVelocity( 0, -0.01, 0, true )
blob.Goto(1)
}
else if( key=="ArrowDown" || revStick.Contains(touchX,touchY) )
{
blob.AddVelocity( 0, 0.01, 0, true )
blob.Goto(5)
}
//Else straighten up blob
else blob.Goto( 3 )
}
//Handle sprite collisions.
function OnCollide( a, b )
{
//console.log( "a:" + a.group + " b:"+b.group )
//Handle character and object collision
if( a.group=="blobs" && b.group=="foods" )
{
//Increase character size
blob.width = blob.width + 0.04 ;
blob.height = blob.height + 0.04 ;
//Play eating sound.
soundEat.Play()
//Vibrate device
gfx.Vibrate( "0,50" );
//Remove food sprite.
gfx.RemoveSprite( b )
}
}